You said you wanted to learn Clarion ⌠Being able to create your own Functions and call them is a common need. Iâll repost the code and the MAP you need to do that âŚ
Iâll keep it simple. My chars have 8 bits, just like .KeepChars() works Bruce. Iâll make them local functions, when that works we can make the APP functions.
#1 - In Embed âLocal Data - Other Declarationsâ put the MAP Prototype declarions. In Clarion you must Declare and Define.
MAP
SortName3x3 PROCEDURE(STRING pFirstName, STRING pLastName),STRING
SortPart4Name PROCEDURE(*STRING pName, BOOL pIsLastName=1, BYTE pLenPart=3),STRING
END
#2 - In Embed âLocal Proceduresâ define your Procedure. Note here the âParametersâ have the RETURN as a ! comment ()!,STRING
because that only goes in the MAP.
SortName3x3 PROCEDURE(STRING pFirstName, STRING pLastName) !,STRING
CODE
RETURN SortPart4Name(pLastName, 1,3) & |
SortPart4Name(pFirstName,0,3)
SortPart4Name PROCEDURE(*STRING pName, BOOL pIsLastName=1, BYTE pLenPart=3) !,STRING
Part STRING(pLenPart) !<-- Sized as specified
P BYTE
X LONG,AUTO
CODE
LOOP X=1 TO SIZE(pName)
CASE pName[X]
OF 'a' to 'z' !First as more common
OROF 'A' to 'Z'
P += 1
Part[P]=pName[X]
IF P=pLenPart THEN BREAK.
END
END
Part=CHOOSE(~pIsLastName,lower(Part),UPPER(Part))
RETURN Part
#3 - In Embed âProcedure Routinesâ
NameCalcs ROUTINE
Cus:FullName=LEFT(CLIP(Cus:FirstName)&' ') & Cus:LastName
DISPLAY(?Cus:FullName)
Cus:SortField=SortName3x3(Cus:FirstName,Cus:LastName)
DISPLAY(?Cus:SortField)
The code you posted earlier had an extra â)â that would cause a compile error.
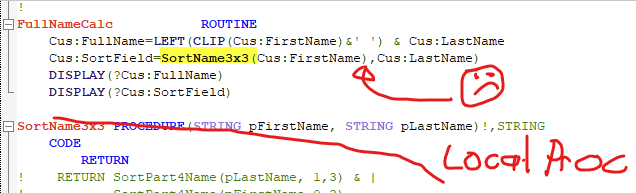
Iâll toss out this code as possibly better for non A-Z alphabets. I donât deal with international issues so YMMV. Read the docs on IsLower(). Edit: change *STRING to no * because PName gets changed.
SortPart4Name PROCEDURE(STRING pName, BOOL pIsLastName=1, BYTE pLenPart=3) !,STRING
Part STRING(pLenPart) !<-- Sized as specified
P BYTE
X LONG,AUTO
CODE
pName=CHOOSE(~pIsLastName,lower(pName),UPPER(pName))
LOOP X=1 TO SIZE(pName)
IF ( pIsLastName AND isUPPER(pName[X])) |
OR (~pIsLastName AND ISlower(pName[X])) THEN
P += 1
Part[P]=pName[X]
IF P=pLenPart THEN BREAK.
END
END
RETURN Part